uvm driver从sequencer FIFO中获取request类型的sequence item(REQ), 类似握手协议, 返回一个response类型的(RSP)。如下图所示:
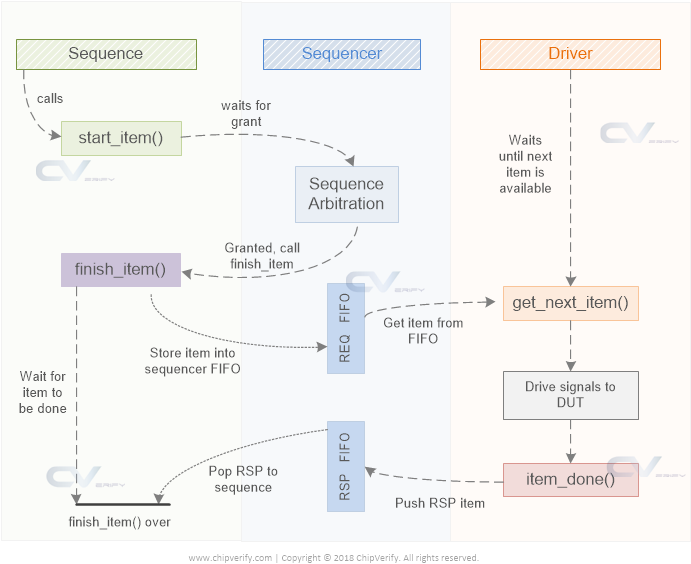
本篇主要介绍在driver中使用get_next_item
的方法。
这种情况下, driver获取sequence item主要是通过seq_item_port
, 比如下面的代码:
1 | class my_driver extends uvm_driver #(my_data); |
一旦driver完成驱动interface上的信号, 就可以用item_done
的方法通知sequencer。
那么sequencer又是如何获取sequence item的呢?
1 | class my_sequence extends uvm_sequence; |