本篇主要介绍uvm环境中的driver。
driver的主要作用是从squencer获取transaction, 然后驱动DUT的interface。如下图所示:
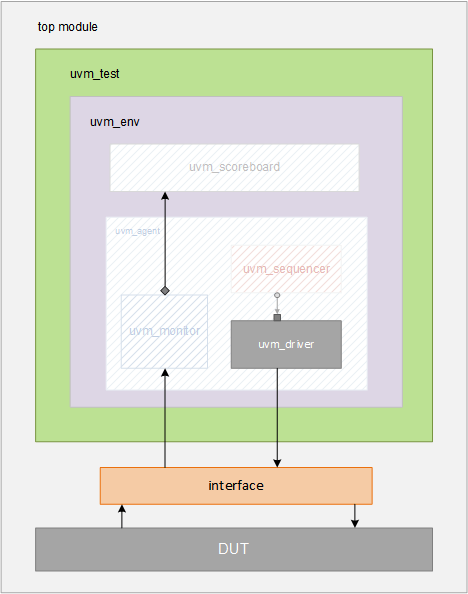
Driver的编写
- 从uvm_driver派生出自定义的class, 比如说是my_driver, 然后用factory机制注册, 并且创建 “new” 函数, 如下面代码所示
1 | // my_driver is user-given name for this class that has been derived from "uvm_driver" |
- 申明 vitual interface, 并在build_phase获取配置:
1 | // Actual interface object is later obtained by doing a get() call on uvm_config_db |
- 编写run_phase
1 | virtual task run_phase (uvm_phase phase); |
Driver-Sequencer 通信
Driver 可以使用TLM端口和sequencer进行通信, 比如uvm_seq_pull_port。driver 可以向squencer请求transaction, 也可以在完成驱动后,进行回应(reponse)。
那么如何使用API呢?
- 在agent或者是env中将sequencer和driver的TLM 端口相连:
1 | virtual function void connect_phase (); |
- 使用握手的方式:get_next_item后面跟item_done();
1 | virtual task run_phase (uvm_phase phase); |
或者是使用下面的方式:
1 | virtual task run_phase (uvm_phase phase); |
示例
下面是一个uvm driver的例子:
1 | class my_driver extends uvm_driver #(my_data); |
参考
本文的图片和code来源于: