本篇主要介绍UVM testbench top和test case如何来写。
test top
testbench 的顶层应该是整个UVM结构的根, 如下图所示, 顶层中可以包含几个test case, test case的下层是XXX_env, env下面又分成几个部门,比如多个uvm_component.
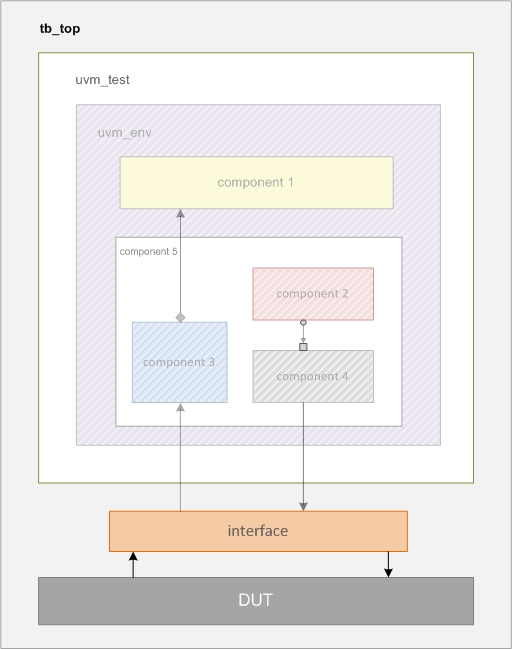
比如是一个testbench 顶层的例子:
1 | module tb_top; |
test case
test case要做的就是这对电路的spec设置不同的激励或者配置,然后检测DUT是否符合设计要求。不同的test case可以设置不同的参数, 但是env可以不必修改。
那么怎么来写一个uvm 的test case呢?
- 使用uvm_test来派生出一个新的class, 比如my_test; 然后使用factory 机制注册, 并写‘new’函数:
1 | class my_test extends uvm_test; |
- 申明env和其他uvm_componet, 并且在build_phase创建实例;
1 | my_env m_top_env; //environment that contains other agents, register models, etc |
- 如果有需要可以打印uvm的拓扑结构
1 | virtual function void end_of_elaboration_phase (uvm_phase phase); |
- 启动sequence, 发送激励
1 |
|
在上文testbench top的介绍中, test case的执行如下:
1 | initial begin |
其中的参数base_test就是test case的名字, 但是实际上可能有很多的test case, 不可能每测试一个case就去修改testbench_top文件, 所以这个参数常常不用给值, 可以在仿真器的命令行中添加:
1 | $> [simulator] -f filelist.f +UVM_TESTNAME=base_test |